100 Days of Code: 51-100
- Kyser Clark
- Dec 31, 2022
- 25 min read
Updated: Jun 4, 2023
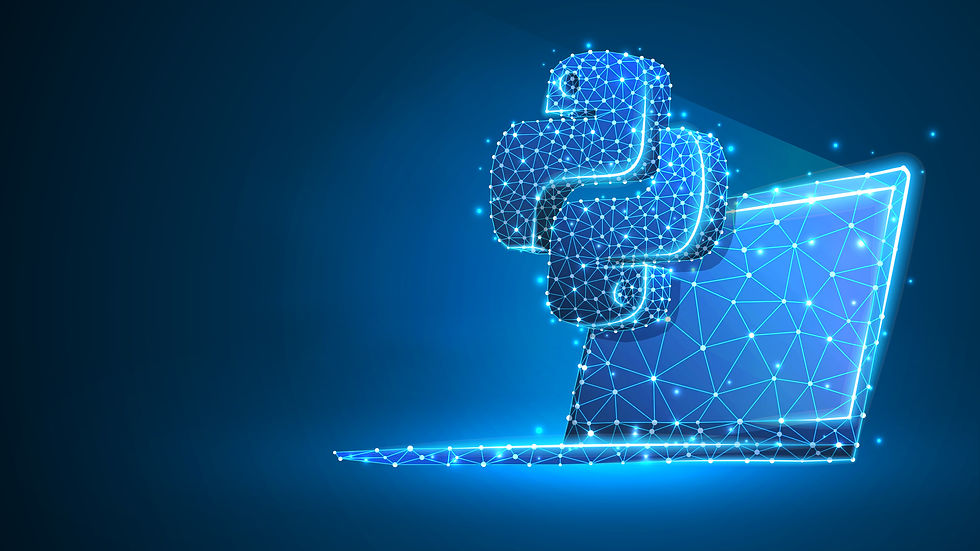
Summary
IT'S DONE!! What a long learning journey that had a lot of ups but also a few downs. The goal was to learn python to be a better hacker and cybersecurity professional. NOT an application developer. And I firmly believe my goal has been accomplished across these 100 days.
The stats:
Total Time Dedicated to Python Learning = 240 hours
5 larger projects
176 small projects
2.2 Books Read
4 video courses completed
2 TryHackMe Rooms
Lessons learned:
I understand how to automate easy but tedious tasks
I can solve simple problems in a programmatic way
I know how to take existing hacking exploits and modify them to work for me (NOT A SCRIPT KIDDIE ANYMORE!)
I understand how to read code, and I can learn how a given program works (for the most part)
I can't build a new robust cybersecurity/hacking tool from the ground up
I am NOT a full fledge application developer, and I have no desire to become one
I must keep coding to make sure I don't get rusty; I plan to do a challenge weekly
I want to learn more programming languages, but not sure if I can dedicate the time to them (learning other skills is going to take priority)
You can view my completed projects at: https://github.com/KyserClark/Completed-Projects
You can view each day's log at: https://github.com/KyserClark/100-Days-of-Code
You can view the Days 1-50 post at: https://www.kyserclark.com/post/100-days-of-code-1-50
Day 100
For the last day of this challenge, I decided to complete a challenge on pythonprinciples.com/challenges/. The challenge I completed was the "Capital Indexes" challenge:
Write a function named capital_indexes. The function takes a single parameter, which is a string. Your function should return a list of all the indexes in the string that have capital letters.
For example, calling capital_indexes("HeLlO") should return the list [0, 2, 4].
My code that solved the challenge:
def capital_indexes(word):
found = []
last_index = -1
for character in word:
if character.isupper() == True:
try:
last_index = word.index(character, last_index+1)
except ValueError:
break
else:
found.append(last_index)
return found
capital_indexes("HeLlO")
The example solutions:
# naive solution
def capital_indexes(s):
upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
result = []
for i, l in enumerate(s):
if l in upper:
result.append(i)
return result
# shorter version
from string import uppercase
def capital_indexes(s):return [i for i in range(len(s)) if s[i] in uppercase]
# you can also use the .isupper() string method.
I'm glad I was able to solve it without the hints, and I'm glad that my code is very different than the intended solutions. Unfortunately, this took me about an hour to complete, so I'll have to continue to do a challenge at least once a week to keep my skills sharp.
Total Time Dedicated to Python Learning = 240 hours
Day 99
Today in one hour, I consolidated all my individual blog posts about this 100 Days of Code journey into two separate blog posts. Transferring 96 blog posts takes a lot of time, but well worth it. I wanted to make a single post, but my website wouldn't let me post for more than 75 days, so I was forced to split the journey into two posts. It was nice re-reading all my old posts and seeing how I progressed throughout this journey.
Total Time Dedicated to Python Learning = 239 hours
Day 98
Today in one hour, I transferred all my python code saved on my computer to my GitHub page. https://github.com/KyserClark/Completed-Projects
Total Time Dedicated to Python Learning = 238 hours
Day 97
Today in one hour, I started and finished the Python for Pentesters room on TryHackMe. Tomorrow I plan on taking all the code I've written across the 100 days and putting it on my GitHub page. After that is complete, I plan on finding daily python practice challenges on the internet to keep my skills sharp.
Total Time Dedicated to Python Learning = 237 hours
Day 96
Today in one hour, I started and finished the Python Basics room on TryHackMe. Tomorrow I will start Python for Pentesters room on TryHackMe.
Total Time Dedicated to Python Learning = 236 hours
Day 95
Today, in one hour, I finished Python 201 for Hackers; I went over Project #5 - Encrypted Bind Shell, Extending Burp, and Debugging. Tomorrow I will start the Python Basics room on TryHackMe.
Total Time Dedicated to Python Learning = 235 hours
Day 94
Today, in one hour, continuing with Python 201 for Hackers, I went over Project #2 - Process Creation and Shellcode Execution (pt1&2), Project #3- Keylogging a System, and Project #4 - Buffer Overflow. Tomorrow I will continue Python 201 for hackers.
Total Time Dedicated to Python Learning = 234 hours
Day 93
Today, in one hour, continuing with Python 201 for Hackers, I went over Pycryptodome, Argparse, and Project #1 - Remote DLL Injection. Tomorrow I will continue Python 201 for hackers.
Total Time Dedicated to Python Learning = 233 hours
Day 92
Today, in one hour, continuing with Python 201 for Hackers I went over Direct Syscalls, Execution From a DLL, BeautifulSoup, Py2exe, Sockets, Scapy, Subprocess, and Threading. Tomorrow I will continue Python 201 for hackers.
Total Time Dedicated to Python Learning = 232 hours
Day 91
Today, in one hour, continuing with Python 201 for Hackers I went over operator overloading, class decorators, introduction to the Windows API, C Data Types and Structures, Interfacing with the Windows API, and Undocumented API Calls. Tomorrow I will continue Python 201 for hackers.
Total Time Dedicated to Python Learning = 231 hours
Day 90
Today, in one hour, I finished Python 101 for Hackers and graduated to Python 201 for Hackers. Today I went over Project #4 - Exploiting a SQL injection, Project #5 - Exploiting a restricted SQL Injection, Decorators, Generators, Serialization, Closures, Inheritance Encapsulation, and Polymorphism. Tomorrow I will continue Python 201 for hackers.
Total Time Dedicated to Python Learning = 230 hours
Day 89
Today is actually a combination of two days. However, I didn't count yesterday because I only put in 30 hours of coding. The 100 Days of Code must be for at least an hour a day. Plus, I counted a 30-minute day earlier in my 100 days of code journey, so it's fair to count both of those half-hour days as one full day.
With that being said, yesterday, I started Python 101 for Hackers. I watched Comprehensions, Lambdas, and Introduction to sys.
Today within an hour, I watched the introduction to requests, introduction to pwntools, Project #1 - SSH login brute forcing, Project #2 - SHA256 password cracking, and Project #3 - Web login form brute forcing. Tomorrow I will Continue with Projects 4 & 5 to close the course.
It's worth noting that I skipped all the basic introductory stuff of the course because I already know the basics of Python and how it works. I wanted to really focus on the content specifically made for hackers.
Total Time Dedicated to Python Learning = 229 hours
Day 88
With 1 hour dedicated to Python coding today, I got through the 3rd section of Chapter 2 in Black Hat Python by Justin Seitz and Tim Arnold. Today was all about building an SSH Server. Once again, unfortunately, the tool did not work as advertised. I carefully read the book, installing every package that it tells me to in the order it tells me to. I coded along and tried my best to understand the code. I even copied and pasted the code from the author's source code library into my machine. Ensuring I change the IP address to my machine's IP address. But no matter what I do, I can't get this SSH server to run. This is the 2nd application in the book that has failed to work. Furthermore, the source code for the book on the NoStarchPress website is a little different than the code in the book. Neither works. It's just frustrating to see this from such a highly-rated book. It's not like I don't know what I'm doing. I know my way around a Linux machine, Python, as well as Hacking concepts VERY well. I honestly think the book is just flawed. Because of this, I am forced to stop reading the book and finish out my last 12 days of Python code using a different learning source.
I will switch to Python 101 for Hackers on the TCM Security Academy website. Once I finish Python 101 for hackers, I will level up to Python 201 for Hackers to close out my 100 days of code.
Total Time Dedicated to Python Learning = 227.5 hours
Day 87
With 1.5 hours dedicated to Python coding today, I got through the 2nd section of Chapter 2 in Black Hat Python by Justin Seitz and Tim Arnold. Today was all about building a TCP Proxy. Unfortunately, the program doesn't work as expected. I double-checked the code and even tested the author's source code, but it does not keep the connection between the local and remote hosts very long. Regardless, it's nice to understand some of the logic that goes into these types of tools.
Total Time Dedicated to Python Learning = 226.5 hours
# I do not take credit for this code. This comes from the book "Black Hat Python 2nd Edition" by Justin Seitz and Tim Arnold
import sys
import socket
import threading
HEX_FILTER = ''.join([(len(repr(chr(i))) == 3) and chr(i) or '.' for i in range(256)])
def hexdump(src, length=16, show=True):if isinstance(src, bytes):
src = src.decode()
results = list()for i in range(0, len(src), length):
word = str(src[i:i+length])
printable = word.translate(HEX_FILTER)
hexa = ' '.join([f'{ord(c):02X}' for c in word])
hexwidth = length*3
results.append(f'{i:04x} {hexa:<{hexwidth}} {printable}')if show:for line in results:print(line)else:return results
def receive_from(connection):
buffer = b""
connection.settimeout(10)try:while True:
data = connection.recv(4096)if not data:break
buffer += data
except Exception as e:print('error ', e)
pass
return buffer
def request_handler(buffer):
# perform packet modifications
return buffer
def response_handler(buffer):
# perform packet modifications
return buffer
def proxy_handler(client_socket, remote_host, remote_port, receive_first):
remote_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
remote_socket.connect((remote_host, remote_port))if receive_first:
remote_buffer = receive_from(remote_socket)if len(remote_buffer):print("[<==] Received %d bytes from remote." % len(remote_buffer))hexdump(remote_buffer)
# remote_buffer = response_handler(remote_buffer)
# client_socket.send(remote_buffer)
# print("[==>] Sent to local.")while True:
local_buffer = receive_from(client_socket)if len(local_buffer):print("[<==] Received %d bytes from local." % len(local_buffer))hexdump(local_buffer)
local_buffer = request_handler(local_buffer)
remote_socket.send(local_buffer)print("[==>] Sent to remote.")
remote_buffer = receive_from(remote_socket)if len(remote_buffer):print("[<==] Received %d bytes from remote." % len(remote_buffer))hexdump(remote_buffer)
remote_buffer = response_handler(remote_buffer)
client_socket.send(remote_buffer)print("[==>] Sent to local.")if not len(local_buffer) or not len(remote_buffer):
client_socket.close()
remote_socket.close()print("[*] No more data. Closing connections.")break
def server_loop(local_host, local_port, remote_host, remote_port, receive_first):
server = socket.socket(socket.AF_INET, socket.SOCK_STREAM)try:server.bind((local_host, local_port))
except Exception as e:print("[!!] Failed to listen on %s:%d" % (local_host, local_port))print("[!!] Check for other listening sockets or correct permissions.")print(e)
sys.exit(0)print("[*] Listening on %s:%d" % (local_host, local_port))
server.listen(5)while True:
client_socket, addr = server.accept()print("> Received incoming connection from %s:%d" % (addr[0], addr[1]))
proxy_thread = threading.Thread(
target=proxy_handler,
args=(client_socket, remote_host,
remote_port, receive_first))
proxy_thread.start()
def main():if len(sys.argv[1:]) != 5:print("Usage: ./proxy.py [localhost] [localport]", end='')print("[remotehost] [remoteport] [receive_first]")print("Example: ./proxy.py 127.0.0.1 9000 10.12.132.1 9000 True")
sys.exit(0)
local_host = sys.argv[1]
local_port = int(sys.argv[2])
remote_host = sys.argv[3]
remote_port = int(sys.argv[4])
receive_first = sys.argv[5]if "True" in receive_first:
receive_first = True
else:
receive_first = False
server_loop(local_host, local_port,
remote_host, remote_port, receive_first)if __name__ == '__main__':main()
Day 86
With two hours dedicated to Python coding today, I got through the first section of chapter 2 in Black Hat Python by Justin Seitz and Tim Arnold. I learned how to create a NetCat-style tool in Python. It works VERY well, and I will undoubtedly use this code when I can't use/install NetCat during a Penetration Test/Red Team engagement but have access to python. I take no credit for this code. Perhaps I can find a way to improve it and make it my own in the future.
Total Time Dedicated to Python Learning = 225 hours
# I do not take credit for this code. This comes from the book "Black Hat Python 2nd Edition" by Justin Seitz and Tim Arnold
import argparse
import socket
import shlex
import subprocess
import sys
import textwrap
import threading
def execute(cmd):
cmd = cmd.strip()if not cmd:return
output = subprocess.check_output(shlex.split(cmd),
stderr=subprocess.STDOUT)return output.decode()class NetCat:
def __init__(self, args, buffer=None):
self.args = args
self.buffer = buffer
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.socket.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
def run(self):if self.args.listen:
self.listen()else:
self.send()
def send(self):
self.socket.connect((self.args.target, self.args.port))if self.buffer:
self.socket.send(self.buffer)try:while True:
recv_len = 1
response = ''while recv_len:
data = self.socket.recv(4096)
recv_len = len(data)
response += data.decode()if recv_len < 4096:breakif response:print(response)
buffer = input('> ')
buffer += '\n'
self.socket.send(buffer.encode())
except KeyboardInterrupt:print('User terminated.')
self.socket.close()
sys.exit()
def listen(self):print('listening')
self.socket.bind((self.args.target, self.args.port))
self.socket.listen(5)while True:
client_socket, _ = self.socket.accept()
client_thread = threading.Thread(target=self.handle, args=(client_socket,))
client_thread.start()
def handle(self, client_socket):if self.args.execute:
output = execute(self.args.execute)
client_socket.send(output.encode())
elif self.args.upload:
file_buffer = b''while True:
data = client_socket.recv(4096)if data:
file_buffer += data
print(len(file_buffer))else:breakwith open(self.args.upload, 'wb') as f:
f.write(file_buffer)
message = f'Saved file {self.args.upload}'
client_socket.send(message.encode())
elif self.args.command:
cmd_buffer = b''while True:try:
client_socket.send(b' #> ')while '\n' not in cmd_buffer.decode():
cmd_buffer += client_socket.recv(64)
response = execute(cmd_buffer.decode())if response:
client_socket.send(response.encode())
cmd_buffer = b''
except Exception as e:print(f'server killed {e}')
self.socket.close()
sys.exit()if __name__ == '__main__':
parser = argparse.ArgumentParser(
description='BHP Net Tool',
formatter_class=argparse.RawDescriptionHelpFormatter,
epilog=textwrap.dedent('''Example:
netcat.py -t 192.168.1.108 -p 5555 -l -c # command shell
netcat.py -t 192.168.1.108 -p 5555 -l -u=mytest.whatisup # upload to file
netcat.py -t 192.168.1.108 -p 5555 -l -e=\"cat /etc/passwd\" # execute command
echo 'ABCDEFGHI' | ./netcat.py -t 192.168.1.108 -p 135 # echo local text to server port 135
netcat.py -t 192.168.1.108 -p 5555 # connect to server
'''))
parser.add_argument('-c', '--command', action='store_true', help='initialize command shell')
parser.add_argument('-e', '--execute', help='execute specified command')
parser.add_argument('-l', '--listen', action='store_true', help='listen')
parser.add_argument('-p', '--port', type=int, default=5555, help='specified port')
parser.add_argument('-t', '--target', default='192.168.1.203', help='specified IP')
parser.add_argument('-u', '--upload', help='upload file')
args = parser.parse_args()if args.listen:
buffer = ''else:
buffer = sys.stdin.read()
nc = NetCat(args, buffer.encode('utf-8'))
nc.run()
Day 85
I finally finished Automate the Boring Stuff with Python by Al Sweigart! I read the rest of chapter 20 and am VERY excited to be done with it. I have officially started the book Black Hat Python by Justin Seitz and Tim Arnold. I completed the introduction sections and chapter 1 which covers virtual machine (VM), Python3, and integrated development environment (IDE) installation. All stuff I'm very familiar with. Chapter 2, Basic Networking Tools, starts getting into the fun stuff. After doing courses and reading books about general python coding (for developers, not hackers), it's nice to finally code stuff specifically for hacking. The real reason why I wanted to begin learning python to begin with. I'm finally excited about the 100 Days of Code journey once again. Automate the Boring Stuff isn't a bad book, but for an aspiring hacker, It was boring. Most of the information didn't apply to the tools I want to write. This is why it took me so long to complete the book, and I stopped coding at the halfway point of the book (and strictly read the book rather than complete the exercises. I'm glad to get excited about my coding journey again and finish my last 15 days strong with a great book specifically tailored for hackers like myself.
Total Time Dedicated to Python Learning = 223 hours
Day 84
Today I read Automate the Boring Stuff with Python by Al Sweigart for an hour. I read the rest of chapter 19 and about half of chapter 20. Chapter 20 is particularly interesting because the book introduced the reader to mouse and keyboard automation. Good for graphical user interfaces (GUIs).
Total Time Dedicated to Python Learning = 222 hours
Day 83
Today I read Automate the Boring Stuff with Python by Al Sweigart for an hour. I read the rest of chapter 18 and about half of chapter 19.
Total Time Dedicated to Python Learning = 221 hours
Day 82
Today I read Automate the Boring Stuff with Python by Al Sweigart for an hour. I read the rest of chapter 17 and about a quarter of chapter 18.
Total Time Dedicated to Python Learning = 220 hours
Day 81
Put in another hour of reading Automate the Boring Stuff with Python by Al Sweigart. I read all of chapters 16 and half of 17.
Total Time Dedicated to Python Learning = 219 hours
Day 80
Put in another hour of reading Automate the Boring Stuff with Python by Al Sweigart. I read all of chapters 14 and 15.
Total Time Dedicated to Python Learning = 218 hours
Day 79
Put in another hour of reading Automate the Boring Stuff with Python by Al Sweigart. Nothing new and exciting here, just another day closer to closing out my goal of 100 days of python.
Total Time Dedicated to Python Learning = 217 hours
Day 78
I took a bit of a break from coding. I'm now back in it and after 1 hour of reading Automate the Boring Stuff with Python by Al Sweigart. I can confidently say that I don't regret the long break, and I still don't really like coding. Development just isn't for me. I have come to the conclusion that I will only code stuff when I find myself repeating long tedious tasks for days on end. Other than that I don't think I will ever develop an entire useful application. I don't have the imagination, desire, or motivation for it. And that is ok because I want to be a hacker, not a developer. I only need to know basic python for my chosen career and I think I'm already past the point I need to know to have a successful ethical hacking career. Maybe things will change when I start to read python books specifically tailored to hacking. Time will tell. I will complete my 100 days of code, and I will finish this book. No matter how much I may not seem to enjoy it.
Total Time Dedicated to Python Learning = 216 hours
Day 77
After over two weeks of not coding, I got back into it. The two-week hiatus was due to a lack of interest in coding, TryHackMe focus shift, contracting covid and being sick, starting a new college course, and a few personal reasons I won't talk about. I'm glad to get back into the saddle.
Today I read all of Chapter 10: Organizing Files in Automate the Boring Stuff with Python by Al Sweigart. I also read about 3/4 of Chapter 11: Debugging. I read all of this in 1 hour's time. For the next day, I will finish Chapter 11, and read Chapter 12: Web Scraping.
Total Time Dedicated to Python Learning = 215 hours
Day 76
In two hours, I read Chapter 8: Input Validation and Chapter 9: Reading and Writing to files in Automate the Boring Stuff with Python by Al Sweigart. Everything in these chapters is something I already knew and already coded out in previous days. Because of that, I chose not to do the practice projects for these chapters. Tomorrow I will start with Chapter 10: Organizing Files.
Total Time Dedicated to Python Learning = 214 hours
Day 75
I took another day off from coding yesterday. This is because I had some personal things to handle. However, it wasn't an entire day off because I still did my daily TryHackMe goal. However, today I managed to squeeze in 2 hours of reading Automate the Boring Stuff with Python by Al Sweigart. I read all of Chapter 7: Pattern Matching with Regular Expressions.
I'll be honest, coding with Python has lost its charm. All the examples in the book and practice projects are programs that don't seem very useful to me. I have no idea how to create a useful program that fixes a problem. I don't want to waste my time coding something no one will use or something that already exists. I understand that it is good practice, but what am I practicing for? Reinventing the wheel? Nonsense. One day I will find a problem that I can solve with code, but I can't think of anything practical that hasn't already been made. So why should I reinvent the wheel with all these practice projects? How does one come up with a good program/application? Something to think about.
Total Time Dedicated to Python Learning = 212 hours
Day 74
Unfortunately, I had to take two days off from 100 Days of Code. I made it 73 days in a row, which I am VERY proud of. I was unable to put time into python the last two days due to a YouTube video that took me about 24 hours to produce and TryHackMe as well as setting my 2022 Goals. I definitely need to streamline my YouTube video creation process. I'm still fairly new to it so I will get better with time. The video looks great by the way. It turned out better than I could imagine. My editing skills are top tier if I say so myself. It can be seen here https://youtu.be/UFBT2Nq4BvI, and it was totally worth taking two days off of 100 Days of Code to make it.
Anyways, back in the saddle again today! In 1.5 hours, I read Chapter 6: Manipulating Strings in Automate the Boring Stuff with Python by Al Sweigart and answered the practice questions. Tomorrow I will code out the two projects: Table Printer, and Zombie Dice Bots, and move on to Part II of the Book: Automating Tasks.
Total Time Dedicated to Python Learning = 210 hours
Day 73
Today was a medium-length day. In four hours I was able to answer Chapter 4's practice questions, and complete the practice projects in Automate the Boring Stuff with Python by Al Sweigart. I then moved on and read the entire length of chapter 5 as well as completing the practice questions and coding out the practice projects, except the chess project, I didn't feel like doing that one in all honesty. It didn't seem as fun as the fanstay_game_inventory. Below is the code for each short project I completed. These were a lot of fun!
Comma Code:
import sys
def comma_code(choice_list): """ Take any python list and print it out without brackets and
separate each index with a comma.""" for object in choice_list: sys.stdout.write(object)if object != choice_list[-1]: sys.stdout.write(', ')
spam = ['apples', 'bananas', 'tofu', 'cats']comma_code(spam)
Coin Flip Streaks:
import random
numberOfStreaks = 0
coin = ['heads', 'tails']
results = []print('Flipping coin 10,000 times...\n')for experimentNumber in range(10_000): # Code that creates a list of 10000 'heads' or 'tails' values. this_flip = (random.choice(coin))print(this_flip)
results.append(this_flip)# Code that checks if there is a streak of 6 heads or tails in a row. if results[-6:] == ['tails'] * 6 or results[-6:] == ['heads'] * 6: numberOfStreaks += 1
percentage = numberOfStreaks / 100print(f'\nNumber of six in a row streaks that happened: {numberOfStreaks}\n')print(f'Chance of having six in a row: {percentage}')
Character Picture Grid:
grid = [['.', '.', '.', '.', '.', '.'],['.', 'O', 'O', '.', '.', '.'],['O', 'O', 'O', 'O', '.', '.'],['O', 'O', 'O', 'O', 'O', '.'],['.', 'O', 'O', 'O', 'O', 'O'],['O', 'O', 'O', 'O', 'O', '.'],['O', 'O', 'O', 'O', '.', '.'],['.', 'O', 'O', '.', '.', '.'],['.', '.', '.', '.', '.', '.']]# Take grid and rotate it 90 degrees to the right and print it on screen
second_list_count = 0for i in range(6): for i in range(9): print(grid[i][second_list_count], end='')
second_list_count += 1 print('')
Fantasy Game Inventory:
# Inventories
stuff = {'rope': 1, 'torch': 6, 'gold coin': 42, 'dagger': 1, 'arrow': 12}
stuff_2 = {'gold coin': 42, 'rope': 1}# Loot of defeated foes
dragon_loot = ['gold coin', 'dagger', 'gold coin', 'gold coin', 'ruby']
troll_loot = ['gold coin', 'spike bat', 'shield', 'troll horn', 'troll horn', 'arrow']def display_inventory(inventory): """ Print out inventory in formatted way. """ print("Inventory:")
item_total = 0 for k, v in inventory.items(): print(f'\t{v} {k}')
item_total += int(v)print("\nTotal number of items: " + str(item_total))def add_to_inventory(inventory, added_items): """ Take defeated foe's loot and add it to inventory. """ for item in added_items: inventory.setdefault(item, 0)
new_item_value = inventory[item] + 1 inventory[item] = new_item_value
add_to_inventory(stuff, troll_loot)display_inventory(stuff)
Total Time Dedicated to Python Learning = 208.5 hours
Day 72
Today I started to read Automate the Boring Stuff with Python by Al Sweigart. With the 6.5 hours, I decided to put towards it today. I managed to read Chapters 1, 2, 3, and 4 (148 pages!). I still need to do the practice questions and practice projects at the end of Chapter 4 though. Tomorrow I hope to read even more of the book. I was feeling off today and I had to do physical therapy as well.
Here is my code for the Collatz sequence:
def collatz(number): if number % 2 == 0: output = (number // 2)else: output = 3 * number + 1 print(output)if output != 1: collatz(output)while True: try: user_number = int(input('Enter Number: '))break except: print('Input must be an integer')collatz(user_number)
Total Time Dedicated to Python Learning = 204.5 hours
Day 71
I have finished the Python Network Programming for Network Engineers Udemy Course by David Bombal. The practical network automation was only about half the course. So I started out with hands-on scriptings and running scripts to apply configurations to network devices. Things the course covered are Netmiko and NAPALM. Unfortunately, I wish there were more practical applications using Netmiko and NAPALM, but the course really only covers the basics. This is how I know I am getting good at python. I put the 2nd half of the course where David Bombal covers the "theory" of Python (Python basics) on 2x speed. This is because I already knew everything he was covered from the previous two courses I completed. This is how I managed to complete this course in such a short period of time (a few days). Next book: Automate the Boring Stuff with Python by Al Sweigart!
Today was a long nine hours making Total Time Dedicated to Python Learning = 198 hours
Day 70
Continuing with the Python Network Programming for Network Engineers Udemy Course by David Bombal, I learned some more automation techniques and added five switches to the topology (see figure 1). I can successfully configure all six switches simultaneously in any manner I choose. Automation is compelling. Unfortunately, I spent the bulk of my time trying to get SSH to work. Every time I tried to SSH from the Network Automation Container to one of the switches, it would not accept my password. After 5-6 hours. I finally discovered that I couldn't SSH into the switches because the Network Automation Container tries to SSH into the root account of the switch by default. That means you have to specify the account you want to SSH to make a connection. And because I wasn't specifying my 'Kyser' account, and I didn't have a 'root' account on the switches, I couldn't make an SSH connection. Once I realized the reason for SSH not working, I rebuilt the entire topology using 'root' as the only account. That way, I don't need to specify a username when I SSH from the Network Automation container to one of the switches. Today was a solid 8.5 hours of labbing. Even though I was getting a bit frustrated about a simple SSH connection not connecting, I had fun. I'm glad I didn't give up and figured it out. I can now continue the course tomorrow. I'm shooting for another 8-9 hours!
figure 1
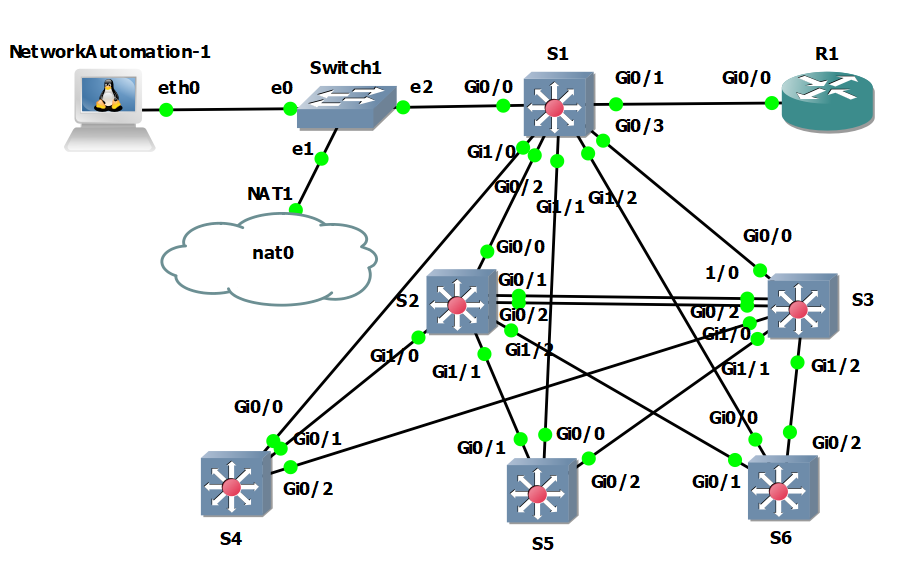
a script that adds n number of VLANs to every switch specified in 'my switches.'
import getpass
import telnetlib
user = input("Enter your telnet username: ")
password = getpass.getpass()
file = open('myswitches')for ip in file: ip = ip.strip()print("Configuring Switch " + ip)
tn = telnetlib.Telnet(ip)
tn.read_until(b"Username: ")
tn.write(user.encode('ascii') + b"\n")if password: tn.read_until(b"Password: ")
tn.write(password.encode('ascii') + b"\n")
tn.write(b"conf t\n")for n in range(2, 20): tn.write(b"vlan " + str(n).encode('ascii') + b"\n")
tn.write(b"name Python_Vlan_" + str(n).encode('ascii') + b"\n")
tn.write(b"end\n")
tn.write(b"exit\n")print(tn.read_all().decode('ascii'))
a script that reads the running-config and saves it to a file for backup for each switch
import getpass
import telnetlib
user = input('Enter your telnet username: ')
password = getpass.getpass()
file = open('myswitches')for ip in file: ip = ip.strip()print('Getting running config from Switch ' + ip)
tn = telnetlib.Telnet(ip)
tn.read_until(b'Username: ')
tn.write(user.encode('ascii') + b'\n')if password: tn.read_until(b'Password: ')
tn.write(password.encode('ascii') + b'\n')
tn.write(b'wr\n')
tn.write(b'terminal length 0\n')
tn.write(b'show run\n')
tn.write(b'exit\n')
readoutput = tn.read_all()
saveoutput = open('switch' + ip, 'w')
saveoutput.write(readoutput.decode('ascii') + '\n')
saveoutput.close()
Total Time Dedicated to Python Learning = 189 hours
Day 69
Continuing with the Python Network Programming for Network Engineers Udemy Course by David Bombal, I made a for loop that can create any number of VLANs on a switch. It was cool making 99 VLANs in a matter of moments. As well as reviewing some python topics I already knew. Only had an hour to put towards Python due to Christmas, but I'm going to hit it hard tomorrow.
Total Time Dedicated to Python Learning = 180.5 hours
Day 68
I DID IT. I automated my first router configuration! Of course, it's a simple script, but it's still automation nonetheless.
Continuing with the Python Network Programming for Network Engineers Udemy Course by David Bombal, I learned how to get Cisco IOS images running in GNS3 (after paying $200 for Cisco Modeling Labs), and I set up a simple topology (see figure 1). Once the topology was created, I made a simple script that configured a loopback address and a basic OSPF configuration on Router 1. The NetworkAutomation computer is a Linux machine that can telnet to devices and run any scripts stored on the device.
Figure 1
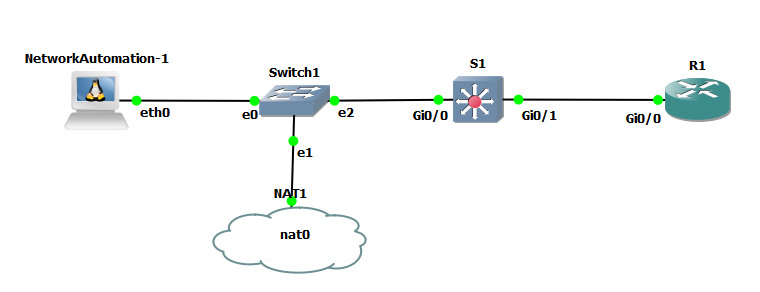
The basic script:
import getpass
import telnetlib
HOST = "192.168.122.71"
user = input("Enter your telnet username: ")
password = getpass.getpass()
tn = telnetlib.Telnet(HOST)
tn.read_until(b"Username: ")
tn.write(user.encode('ascii') + b"\n")if password: tn.read_until(b"Password: ")
tn.write(password.encode('ascii') + b"\n")
tn.write(b"enable\n")
tn.write(b"Kyser\n")
tn.write(b"conf t\n")
tn.write(b"int loop 0\n")
tn.write(b"ip add 1.1.1.1 255.255.255.255 \n")
tn.write(b"router ospf 1 \n")
tn.write(b"network 0.0.0.0 255.255.255.255 area 0 \n")
tn.write(b"end\n")
tn.write(b"exit\n")print(tn.read_all().decode('ascii'))
Total Time Dedicated to Python Learning = 179.5 hours
Day 67
Continuing along with the Python Network Programming for Network Engineers Udemy Course by David Bombal, I discovered the free Cisco Dev Net Labs usable for the course. However, I ran into many issues with this free solution. The free Dev Net Labs require Cisco any connect to run. I had a lot of problems with the Cisco Any Connect VPN. I was forced to restart my computer a few times, and the one time I did get it working, any connect disconnected a few minutes into my lab. With this in mind, I don't want to be randomly kicked off in the middle of my labs during this course, so I think I will purchase full image access from Cisco. $200 is a lot of money for a lab, but when it comes to my career as a Network Engineer, it's 100% worth it. Tomorrow I will complete the purchase and finally get into real network automation.
Total Time Dedicated to Python Learning = 177 hours
Day 66
I didn't do actual coding today. Instead, I spent over an hour installing GNS3 and the GNS3 VM to follow along with Python Network Programming for Network Engineers Udemy Course by David Bombal.
Unfortunately, the Cisco IOS images I need for the course cost $200 and only grants 1-year access. This is a very steep and significant cost, especially since it's only good for 365 Days. I will sleep on the decision if I want to purchase them or not. I may do it; I may not. If I decide not to buy the images, I will proceed with a different Python course. However, I am most interested in Python for Network Engineers since I am not a developer. I am a Network Engineer and Cybersecurity professional. Therefore, this course will be more beneficial than most other Python courses.
Total Time Dedicated to Python Learning = 175.75 hours
Day 65
I finally finished Python Crash Course by Eric Matthes! Once I finished it I immediately began the Python Network Programming for Network Engineers Udemy Course by David Bombal. I spent 2 hours trying to get GNS3 to work with Virtual Box. No success. I will try again tomorrow. If I am unable to get GNS3 working I will have to start a different Python course.
Total Time Dedicated to Python Learning = 174.25 hours
Day 64
Put in three solid hours today. I finished Chapter 19: User Accounts in Python Crash Course by Eric Matthes and continued about a third of the way on to Chapter 20: Styling and Deploying an App. I'll be honest, I stopped coding in the last section of Chapter 19. From this point on I will only be reading the book and not coding along. This is because I officially lost all desire to learn web development. A simple website takes so many lines of code, it's insane. It's not fun to me and I can't grasp my head around everything the author is throwing at me at this point. My goal is to finish reading the book and hope that at least some of the information sticks in my brain. My goal is to be a hacker. Not a web developer. I only need to know enough to break into websites, not build them from scratch. The thief knows how to infiltrate the castle and take what he/she wants. The thief doesn't need to know how every stone was placed, as the thief only needs a single way in and a single way out.
Total Time Dedicated to Python Learning = 171.25 hours
Day 63
Got a late start on the day due to YouTube video editing. I wanted to complete both Chapter 19 and 20 in Python Crash Course by Eric Matthes. However, I was only able to get an hour of coding in and I only managed to get about a quarter the way through Chapter 19: User Accounts. The web application is in a working state with slightly more functionality for users to add topics and entries.
My work is attached:
Total Time Dedicated to Python Learning = 168.25 hours
Day 62
I Logged a very nice six hours into Python learning today. I made a lot of progress in Python Crash Course by Eric Matthes. I started out by finishing up Chapter 16: Downloading Data and completing Chapter 17: Working with APIs. Then moving on to Project 3: Web Applications. I started and finished Chapter 18: Getting Started with Django. Tomorrow I will complete chapter 19: User Accounts and Chapter 20: Styling and Deploying an App.
My work is attached:
Total Time Dedicated to Python Learning = 167.25 hours
Day 61
Today in four hours I completed Chapter 15: Generating Data in Python Crash Course by Eric Matthes. I continued and completed half of Chapter 16: Downloading Data. Tomorrow I will complete Chapter 16 and move on to Chapter 17: Working with APIs.
All my Data Visualization work up to this point is attached:
Total Time Dedicated to Python Learning = 161 hours
Day 60
I have completed the Alien Invasion Game. I will admit, the code is not all mine. It's not a copy and paste. However, I did type it ALL out manually, following the project in Python Crash Course by Eric Matthes step by step. I changed a few things about the game to make it my own, but not much. I learned a lot about game development in the past four days. I have a newfound respect for game developers. This code is a remake of a game developed in 1978 and it's ridiculous how much coding goes into such a simple game. I have concluded that I will never develop anything worth playing, and that's ok in my book. I'm a cybersecurity professional, not a game developer. Once I completed Chapter 14: Scoring, I moved on to Project 2: Data Visualization. Probably more useful for my career than game development. I made it about a quarter of the way through Chapter 15: Generating Data.
The complete Alien Invasion Game is attached:
As well as my first data visualization charts:
Total Time Dedicated to Python Learning = 157 hours
Day 59
Today I logged three hours into the Alien Invasion Game project. I finished Chapter 12: Aliens! in Python Crash Course by Eric Matthes. First, I started with making the alien fleet move, then detected bullet-alien collisions and removed any alien hit by a bullet. Next, I added end game conditions when the player ship is hit by an alien or if an alien drops off the bottom of the screen and sets the game to 3 lives. Then I started Chapter 14: Scoring, where the "Play" button was created to start the game. Then I made increasing difficulty levels for each time the alien fleet was wiped. The game speeds up after completing each level. Tomorrow I begin the "Scoring" section of the chapter where the game's scoring system is implemented.
The current working game files are attached:
Total Time Dedicated to Python Learning = 154 hours
Day 58
I logged three hours today. I finished Chapter 12: A Ship the Fires Bullets in Python Crash Course by Eric Matthes. I limited the ship's range so it couldn't fly off the screen; I added a 'Q' to quit control, made the game run in fullscreen, made the ship fire bullets, and limited the player to only three bullets on screen, once to promote accuracy. Once the ship was able to shoot bullets it was time to build a fleet of Aliens in Chapter 13: Aliens! At its time, the game has an entire fleet of aliens that do not move and do not disappear when hit by a bullet. Tomorrow that will change when I continue and finish chapter 13.
The current working game files are attached:
Total Time Dedicated to Python Learning = 151 hours
Day 57
I continued Chapter 12: A Ship the Fires Bullets in Python Crash Course by Eric Matthes. I resized the ship and made it go to the bottom of the screen instead of the top left. I also coded the left and right controls to move the ship left and right. Lastly, I made a setting to adjust the shipping speed if needed. Tomorrow I will continue and finish chapter 12. The current game state is attached:
Total Time Dedicated to Python Learning = 148 hours
Day 56
I Started the Alien Invasion Game Project in Python Crash Course by Eric Matthes; today, I created a pygame window that responds to user input, set the background color, created a settings class, and added the ship image to the game window by creating the ship class and drawing the ship to the screen. Tomorrow I will continue with the project.
My game code is attached. You can run alien_invasion.py on your computer and see the current status of the game!
Total Time Dedicated to Python Learning = 147 hours
Day 55
Today I started Chapter 12: A Ship That Fires Bullets in in Python Crash Course by Eric Matthes. Early in the chapter, the author mentions that you should use Git for version control for the Alien Invasion game. Since I'm very new to Git, I decided to spend today reading Appendix D: Using Git for Version control and following along with the "tutorial" by installing Git and going through the basic commands on my own computer. I finished my coding session by installing Pygame. Tomorrow I will continue chapter 12 and officially start coding the Alien Invasion game.
Total Time Dedicated to Python Learning = 145.5 hours
Day 54
Today I finished Chapter 11: Testing Your Code in Python Crash Course by Eric Matthes. It took me about an hour and a half to read the rest of the chapter and complete the last exercise. To be honest, I'm not sure of the advantages of the test methods presented in the book over my previous testing method of "trial and error." It seems as if the test exercises served no real advantage over running the code a few times and looking at the 'real' results. This was all new content to me, as this way of testing was never mentioned in my previous Udemy course, so I wonder how other Python developers feel about unittest. Chapter 11 completes Part I of the book, and tomorrow I will start Part II: Projects. There are three projects in Part II. Alien Invasion: Making a Game with Python, Data Visualization, and Web Applications. I have elected to do the projects in order, so I will start with Chapter 12: A Ship That Fires Bullets.
My exercises from the book are attached:
Total Time Dedicated to Python Learning = 144.5 hours
Day 53
Great Day of Python! I haven't put in over 2 hours for a while. Today I logged 2.5 hours into Python. I first started out the day by switching my IDE back to PyCharm. I do like it more than Microsoft VS Code. I finished Chapter 10: Files and Exceptions in Python Crash Course by Eric Matthes. And started Chapter 11: Testing Your Code. I'm learning tests that I didn't even know you could do, so that is very nice. Tomorrow I will continue and finish Chapter 11.
My exercises from the book are attached:
Total Time Dedicated to Python Learning = 143 hours
Day 52
Another hour of Python learning today! I almost finished Chapter 10: Files and Exceptions in Python Crash Course by Eric Matthes. I just need to program the three exercises and read the summary at the end of the chapter, and then I can move on to Chapter 11: Testing Your Code. I'm getting pretty excited because I am getting closer to the fun projects for the course.
My exercises from the book are attached:
Total Time Dedicated to Python Learning = 140.5 hours
Day 51
Today with the 1.5 hours I had, I continued Chapter 10: Files and Exceptions in Python Crash Course by Eric Matthes. I spent some time familiarizing myself with Microsoft VS Code and wrote some simple programs dealing with exceptions while opening files.
My exercises from the book are attached:
Total Time Dedicated to Python Learning = 139.5 hours